API call code snippet
This page contains a code snippet of an elementary call to MT Companion Machine Translation API and the authentication using Azure AD.
How to get the access token from Azure AD
When you are using API calls of MT Companion, you need to get the access token first. Now the Azure AD must be called before any of the MT Companion API POST call with following parameters (cURL format):
curl --location --request
POST 'https://login.microsoftonline.com/ead220ab-1743-4c57-83ae-e055f3401f19/oauth2/v2.0/token' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'client_id={YOUR CLIENT ID GOES HERE}' \
--data-urlencode 'client_secret={YOUR CLIENT SECRET GOES HERE}' \
--data-urlencode 'grant_type=client_credentials' \
--data-urlencode 'scope=https://mtcompanion.rwsworldwide.com/.default'
Note that GUID code ead220ab-1743-4c57-83ae-e055f3401f19
in the request
URI represents RWS Azure tenant.
Response from this call contains token information including the access token:
{
"token_type": "Bearer",
"expires_in": 3599,
"access_token": "eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsIng1d..."
}
Postman example:
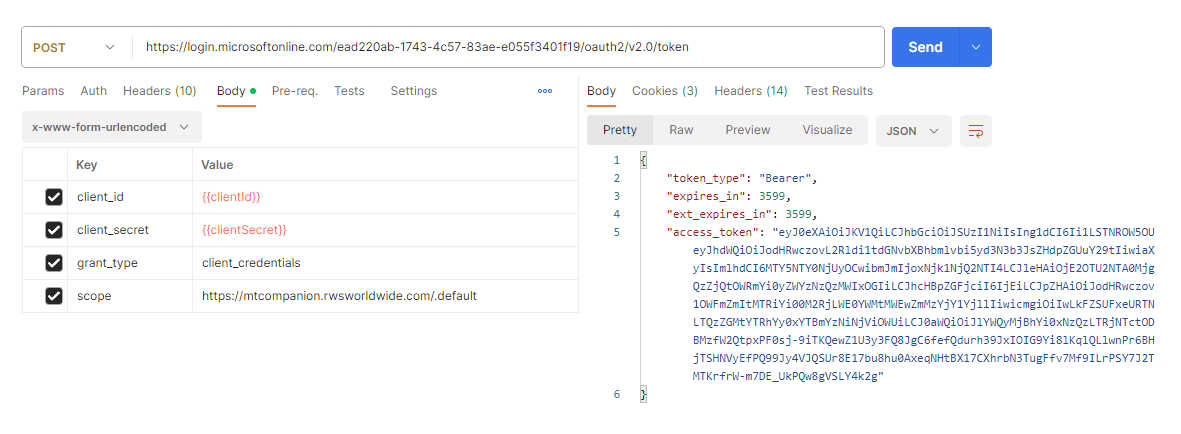
Complete code in C#
class Program
{
//All parameters in constants:
const string AzureTenantId = "ead220ab-1743-4c57-83ae-e055f3401f19";
const string AzureClientId = "{{clientId}}";
const string AzureClientSecret = "{{clientSecret}}";
const string AzureGrantType = "client_credentials";
const string AzureScope = "https://mtcompanion.rwsworldwide.com/.default";
const string MtCompanionTranslateUrl = "https://companion.languageweaver.com/api/v4/mt/translate";
// ------------------------------------------------------------------------------------------------
static void Main(string[] args)
{
string[] stringArray = { "This is a test." };
var azureToken = GetToken();
var translateOutput = GetTranslation(
azureToken.token_type, azureToken.access_token,
"MK_MS", "EN", "CS", "xml",
stringArray);
Console.WriteLine(translateOutput.units[0].translation);
}
// ------------------------------------------------------------------------------------------------
private static TokenStruct GetToken()
{
var azureUrl = $"https://login.microsoftonline.com/{AzureTenantId}/oauth2/v2.0/token";
var content = new FormUrlEncodedContent(
new List<KeyValuePair<string, string>>
{
new("client_id", AzureClientId),
new("client_secret", AzureClientSecret),
new("grant_type", AzureGrantType),
new("scope", AzureScope)
});
var mediaType = new MediaTypeWithQualityHeaderValue("application/json");
using var client = new HttpClient();
client.DefaultRequestHeaders.Accept.Add(mediaType);
var responseAzure = client.PostAsync(new Uri(azureUrl).AbsoluteUri, content).Result;
return responseAzure.IsSuccessStatusCode
? responseAzure.Content.ReadFromJsonAsync<TokenStruct>().Result
: null;
}
// ------------------------------------------------------------------------------------------------
private static ResponseStringArray GetTranslation(
string tokenType, string tokenValue,
string configAlias, string langFrom, string langTo, string textFormat,
string[] arrSources)
{
var mediaType = new MediaTypeWithQualityHeaderValue("application/json");
var authHeader = new AuthenticationHeaderValue(tokenType, tokenValue);
using var client = new HttpClient();
client.DefaultRequestHeaders.Accept.Add(mediaType);
client.DefaultRequestHeaders.Authorization = authHeader;
var responseMtc = client.PostAsJsonAsync<string[]>(
MtCompanionTranslateUrl +
$"?configAlias={configAlias}&from={langFrom}&to={langTo}&textFormat={textFormat}",
arrSources).Result;
return responseMtc.IsSuccessStatusCode
? responseMtc.Content.ReadFromJsonAsync<ResponseStringArray>().Result
: null;
}
}
// --------------------------------------------------------------------------------------------------
internal class TokenStruct
{
public string access_token { get; set; }
public long expires_in { get; set; }
public long ext_expires_in { get; set; }
public string token_type { get; set; }
};
internal class ResponseStringArray
{
public string jobId { get; set; }
public DateTime created { get; set; }
public string configurationAlias { get; set; }
public string sourceCode { get; set; }
public string targetCode { get; set; }
public string textFormat { get; set; }
public JobStatusCode statusCode { get; set; }
public string statusMessage { get; set; }
public Unit[] units { get; set; }
}
internal class Unit
{
public string source { get; set; }
public string translation { get; set; }
public bool isFromCache { get; set; }
}
internal enum JobStatusCode
{
Undefined = 0,
Done = 1,
DoneWithIgnoredErrors = 2,
Failed = 3
}